Scripts
Recommended Scripts
Automatic LCDs 2
Everything you will ever need to know about your ship and station displayed in real time on LCD panels. Includes cockpit panel support! This advanced script is typically favored and recommended for ALL types of VSF grids.
➕ Subscribe on Steam 📎 View custom VSF snippets
Fancy Status Displays V2
A more visual, but less versatile version of Automatic LCDs 2. Excellent for creating large, easy-to-read status bars.
Isy’s Inventory Manager
A vital script that supports a myriad of features from component quotas to cargo sorting. This script is highly recommended for all VSF grids, as having it active will prevent our Federation stations from interfering with your local cargo holds when you dock to them.
Isy’s Cargo Bars
An incredibly neat, lean script that projects the current fill level of all cargo containers onto adjacent LCD’s.
PAM – Path Auto Miner
An extremely effective and popular script for fully automating the ore acquisition process. The script will do everything from docking and undocking from your station, to moving to pre-assigned asteroid locations and automatically stripping them of their ore.
Rdav’s Fleet Command MkII
A remarkably advanced script that allows for RTS-style movement of “drones” grids to perform a huge list of tasks. This one comes with the ability to use the software on an LCD with full cursor support.
Whips Radar (custom modified)
An essential script that shows a visual overview of your surroundings, as well as a list of all enemy and friendly units around you. This is critical for PVP, as enemy players can lurk and follow you into warp if you fail to notice them.
📎 View snippet in the snippet library
SIMPL | Ship Integrity Monitoring Program Lite
This script will visually show damage and vital systems on an easy-to-read LCD that you ideally place close to your cockpit. It will print out real-time changes as your ship encounters enemies so you can adapt to the situation appropriately.
Snippet Library
Snippets for the
Expanse Live Map
This snippet of code will create a live map to help you traverse the Draconis Expanse server. This is provided with the starter ships.
Placeholder
Placeholder
string _mapLCD = “LCD Panel [System Map]”; // LCD Name
string _shipImage = “DrewRazerback”; // DrewRazerback
int _shipScaleFull = 30; // Change Size if needed
int _shipScaleZoomed = 45; // Change Size if needed
// – Configuration above – Do not touch below –
Vector3D _gpsCoordinates = Vector3D.Zero;
IMyCockpit _cockpit;
IMyTextPanel _lcd;
IMyTextSurface _drawingSurface;
RectangleF _viewport;
MySprite _backgroundSprite;
string _currentMap;
int _lcdOffsetLeft;
int _lcdOffsetTop;
bool _zoomed;
bool _enabled = true;
int _drawCounter = 0;
int _enabledTicks = 0;
public Program()
{
_cockpit = GridTerminalSystem.GetBlockWithName(_mapCockpit) as IMyCockpit;
if (_cockpit == null)
{
Echo($”Cockpit not defined. MapCockpit: {_mapCockpit}”);
return;
}
// Step 4: Check if the LCD is defined
_lcd = GridTerminalSystem.GetBlockWithName(_mapLCD) as IMyTextPanel;
if (_lcd == null)
{
Echo($”LCD not defined. MapLCD: {_mapLCD}”);
return;
}
_lcd.Enabled = _enabled;
_drawingSurface = GridTerminalSystem.GetBlockWithName(_mapLCD) as IMyTextSurface;
// Set the continuous update frequency of this script
Runtime.UpdateFrequency = UpdateFrequency.Update100;
_viewport = new RectangleF(
(_drawingSurface.TextureSize – _drawingSurface.SurfaceSize) / 2f,
_drawingSurface.SurfaceSize
);
_zoomed = false;
// Make the text surface display sprites
PrepareTextSurfaceForSprites(_drawingSurface);
}
public void Save()
{
// Called when the program needs to save its state. Use
// this method to save your state to the Storage field
// or some other means.
//
// This method is optional and can be removed if not
// needed.
}
public void Main(string argument, UpdateType updateSource)
{
if (_lcd == null) { Echo(“LCD not found”); return; }
if (!string.IsNullOrWhiteSpace(argument))
{
if (argument.Equals(“zoom”, StringComparison.CurrentCultureIgnoreCase))
{
_zoomed = !_zoomed;
}
else
{
if (argument.Equals(“toggle”, StringComparison.CurrentCultureIgnoreCase))
{
_enabled = !_enabled;
}
else if (argument.Equals(“off”, StringComparison.CurrentCultureIgnoreCase))
{
_enabled = false;
}
else if (argument.Equals(“enable”, StringComparison.CurrentCultureIgnoreCase))
{
_enabled = true;
}
_lcd.Enabled = _enabled;
}
}
if (_enabled) { _enabledTicks++; }
if (!_enabled || _enabledTicks > 500)
{
Echo(“Disabled, run with ‘toggle’ to turn on”);
_lcd.Enabled = false;
_enabledTicks = 0;
_enabled = false;
return;
}
// Begin a new frame
var frame = _drawingSurface.DrawFrame();
// All sprites must be added to the frame here
DrawSprites(ref frame);
// We are done with the frame, send all the sprites to the text panel
frame.Dispose();
}
public void DrawSprites(ref MySpriteDrawFrame frame)
{
_drawCounter++;
if (_drawCounter % 10 == 0)
{
//frame.Add(new MySprite()
//{
// Type = SpriteType.TEXTURE,
// Data = “”,
// Position = _viewport.Center,
// Size = _viewport.Size,
// Color = Color.White.Alpha(0.66f),
// Alignment = TextAlignment.CENTER
//});
frame.Add(new MySprite());
_drawCounter = 0;
}
_gpsCoordinates = GetGPSCoordinates(_cockpit);
int gpsX = (int)Math.Round(_gpsCoordinates.X);
int gpsY = (int)Math.Round(_gpsCoordinates.Y);
int gpsZ = (int)Math.Round(_gpsCoordinates.Z);
Echo($”GPS X: {gpsX}”);
Echo($”GPS Y: {gpsY}”);
Echo($”GPS Z: {gpsZ}”);
var maxGPS = 10000000m;
_lcdOffsetLeft = 0;
_lcdOffsetTop = 10;
var lcdXAxisOffset = 256;
var lcdYAxisOffset = 256;
var gpsXOffset = 0;
var gpsYOffset = 0;
var shipSize = new Vector2(_shipScaleFull, _shipScaleFull);
if (!_zoomed) { _currentMap = “ExpanseNewMap”; }
else
{
maxGPS = maxGPS / 2;
shipSize = new Vector2(_shipScaleZoomed, _shipScaleZoomed);
if (gpsX > 0 && gpsY > 0) { _currentMap = “ExpanseMapTR”; gpsXOffset = (int)(-1 * maxGPS / 2); gpsYOffset = (int)(-1 * maxGPS / 2); }
else if (gpsX > 0) { _currentMap = “ExpanseMapBR”; gpsXOffset = (int)(-1 * maxGPS / 2); gpsYOffset = (int)(maxGPS / 2); }
else if (gpsY > 0) { _currentMap = “ExpanseMapTL”; gpsXOffset = (int)(maxGPS / 2); gpsYOffset = (int)(-1 * maxGPS / 2); }
else { _currentMap = “ExpanseMapBL”; gpsXOffset = (int)(maxGPS / 2); gpsYOffset = (int)(maxGPS / 2); }
}
var gpsPerPixel = maxGPS / 512m;
Echo($”GPS Per Pixel:{gpsPerPixel}”);
_backgroundSprite = new MySprite()
{
Type = SpriteType.TEXTURE,
Data = _currentMap,
Position = _viewport.Center,
Size = _viewport.Size,
Color = Color.White.Alpha(0.66f),
Alignment = TextAlignment.CENTER
};
frame.Add(_backgroundSprite);
Echo($”Viewport: {_viewport.Size}”);
var lcdMin = 12;
var lcdMax = 500;
var lcdEdge = false;
int lcdX = (int)((gpsX + gpsXOffset) / gpsPerPixel + lcdXAxisOffset + _lcdOffsetLeft);
int lcdY = (int)(-1 * (gpsY + gpsYOffset) / gpsPerPixel + lcdYAxisOffset + _lcdOffsetTop);
// Clamp inside lcd
if (lcdX >= lcdMax) { lcdEdge = true; lcdX = lcdMax; }
else if (lcdX <= lcdMin) { lcdEdge = true; lcdX = lcdMin; }
if (lcdY >= lcdMax) { lcdEdge = true; lcdY = lcdMax; }
else if (lcdY <= lcdMin) { lcdEdge = true; lcdY = lcdMin; }
Echo($"LCD X: {lcdX}");
Echo($"LCD Y: {lcdY}");
Echo($"Over Edge: {lcdEdge}");
var position = new Vector2(lcdX, lcdY);
var angle = GetAngle(_cockpit);
var sprite = new MySprite()
{
Type = SpriteType.TEXTURE,
Data = _shipImage,
Position = position,
Size = shipSize,
RotationOrScale = -1 * ((float)angle - 1.5f),
Color = Color.White.Alpha(0.66f),
Alignment = TextAlignment.CENTER
};
frame.Add(sprite);
}
public Vector3D GetGPSCoordinates(IMyCockpit cockpit)
{
return cockpit.GetPosition();
}
public double GetAngle(IMyCockpit cockpit)
{
// Get the cockpit's orientation in world space
MatrixD orientation = cockpit.WorldMatrix;
// Get the forward vector
Vector3D forward = orientation.Forward;
// Calculate the angle based on forward.X and forward.Y
double angle = Math.Atan2(forward.Y, forward.X);
// Convert the angle to degrees
double angleDegrees = angle * 180 / Math.PI;
return angle;
}
public void PrepareTextSurfaceForSprites(IMyTextSurface textSurface)
{
// Set the sprite display mode
textSurface.ContentType = ContentType.SCRIPT;
// Make sure no built-in script has been selected
textSurface.Script = "";
}[/et_pb_accordion_item][/et_pb_accordion][/et_pb_column][et_pb_column type="2_5" _builder_version="4.19.4" _module_preset="default" global_colors_info="{}"][dipi_image_hotspot img_src="data:image/svg+xml;base64,PHN2ZyB3aWR0aD0iMTA4MCIgaGVpZ2h0PSI1NDAiIHZpZXdCb3g9IjAgMCAxMDgwIDU0MCIgeG1sbnM9Imh0dHA6Ly93d3cudzMub3JnLzIwMDAvc3ZnIj4KICAgIDxnIGZpbGw9Im5vbmUiIGZpbGwtcnVsZT0iZXZlbm9kZCI+CiAgICAgICAgPHBhdGggZmlsbD0iI0VCRUJFQiIgZD0iTTAgMGgxMDgwdjU0MEgweiIvPgogICAgICAgIDxwYXRoIGQ9Ik00NDUuNjQ5IDU0MGgtOTguOTk1TDE0NC42NDkgMzM3Ljk5NSAwIDQ4Mi42NDR2LTk4Ljk5NWwxMTYuMzY1LTExNi4zNjVjMTUuNjItMTUuNjIgNDAuOTQ3LTE1LjYyIDU2LjU2OCAwTDQ0NS42NSA1NDB6IiBmaWxsLW9wYWNpdHk9Ii4xIiBmaWxsPSIjMDAwIiBmaWxsLXJ1bGU9Im5vbnplcm8iLz4KICAgICAgICA8Y2lyY2xlIGZpbGwtb3BhY2l0eT0iLjA1IiBmaWxsPSIjMDAwIiBjeD0iMzMxIiBjeT0iMTQ4IiByPSI3MCIvPgogICAgICAgIDxwYXRoIGQ9Ik0xMDgwIDM3OXYxMTMuMTM3TDcyOC4xNjIgMTQwLjMgMzI4LjQ2MiA1NDBIMjE1LjMyNEw2OTkuODc4IDU1LjQ0NmMxNS42Mi0xNS42MiA0MC45NDgtMTUuNjIgNTYuNTY4IDBMMTA4MCAzNzl6IiBmaWxsLW9wYWNpdHk9Ii4yIiBmaWxsPSIjMDAwIiBmaWxsLXJ1bGU9Im5vbnplcm8iLz4KICAgIDwvZz4KPC9zdmc+Cg==" _builder_version="4.19.4" _module_preset="default" custom_padding="|20px||20px|false|false" global_colors_info="{}"][/dipi_image_hotspot][/et_pb_column][/et_pb_row][et_pb_row column_structure="3_5,2_5" admin_label="Row" _builder_version="4.19.4" _module_preset="default" background_color="#FFFFFF" width_tablet="" width_phone="100%" width_last_edited="on|phone" custom_margin="||||false|false" custom_padding="|30px||30px|false|false" global_colors_info="{}"][et_pb_column type="3_5" _builder_version="4.19.4" _module_preset="default" global_colors_info="{}"][et_pb_text admin_label="NavOS" _builder_version="4.27.0" _module_preset="default" custom_padding="|15px||15px|false|false" global_colors_info="{}"]
NavOS 2 (for Draconis Expanse)
This snippet of code will allow you to “turn and burn”, as well as automatically fly to destinations with provided GPS points. It is rumored to be an edited version of the workshop variant.
[/et_pb_text]Placeholder
Click to reveal code
Script Not Found.

Whip’s Retro Thruster Braking Script v5
This is a lean alternative to NavOS 2 that simply allows for a quick “turn and burn”.
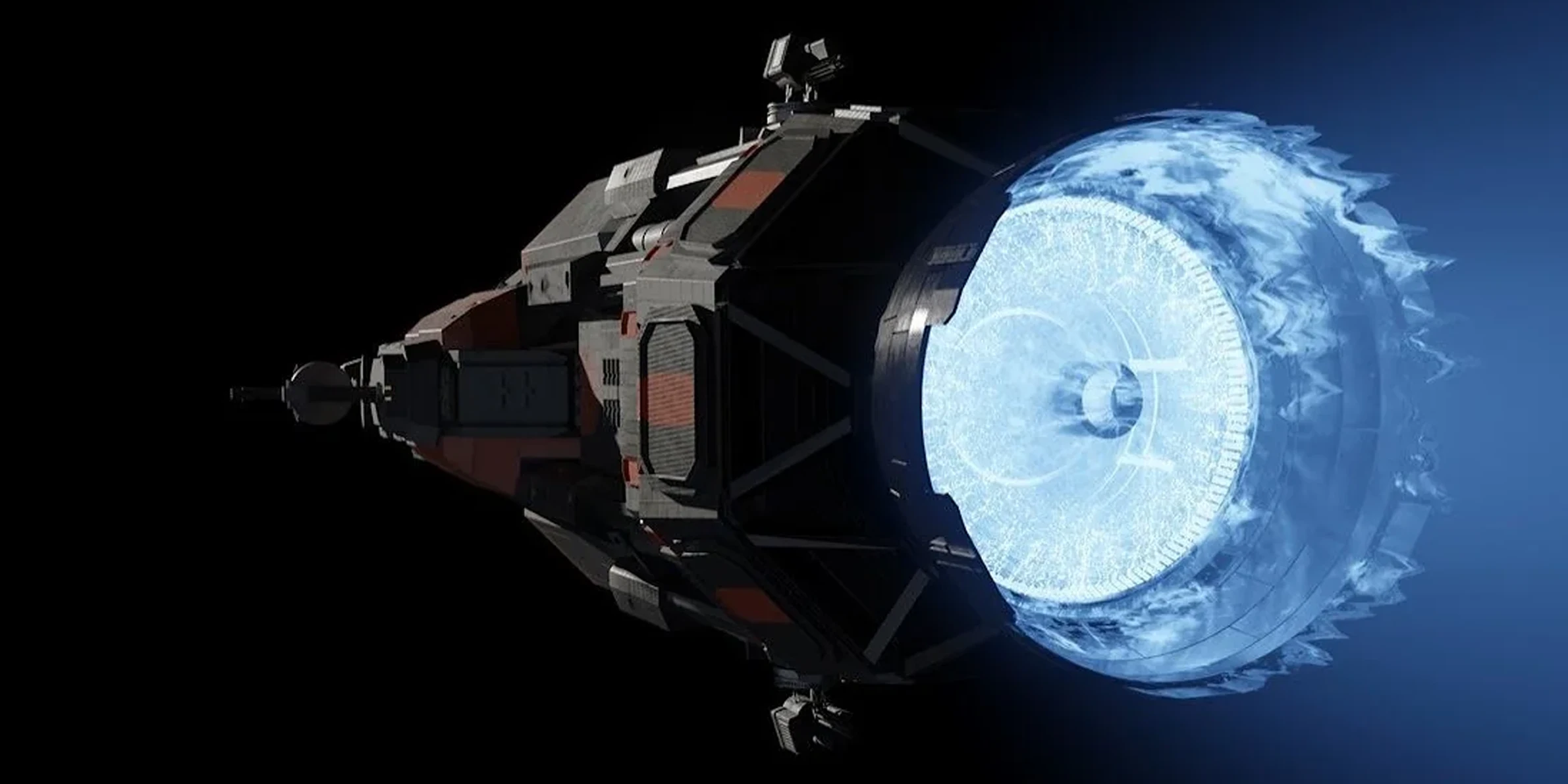
Snippet for
Whips Radar Script (Modified)
Place this snippet into a programming block under the “edit” tab. Then, place “Radar” into the name of an LCD you’d like to use as a visual radar. Additionally, place “Target LCD” into the name of any LCD you’d like to use for your target list system. This script also allows for an automatic audio and visual (lights) alert when enemies are first detected.
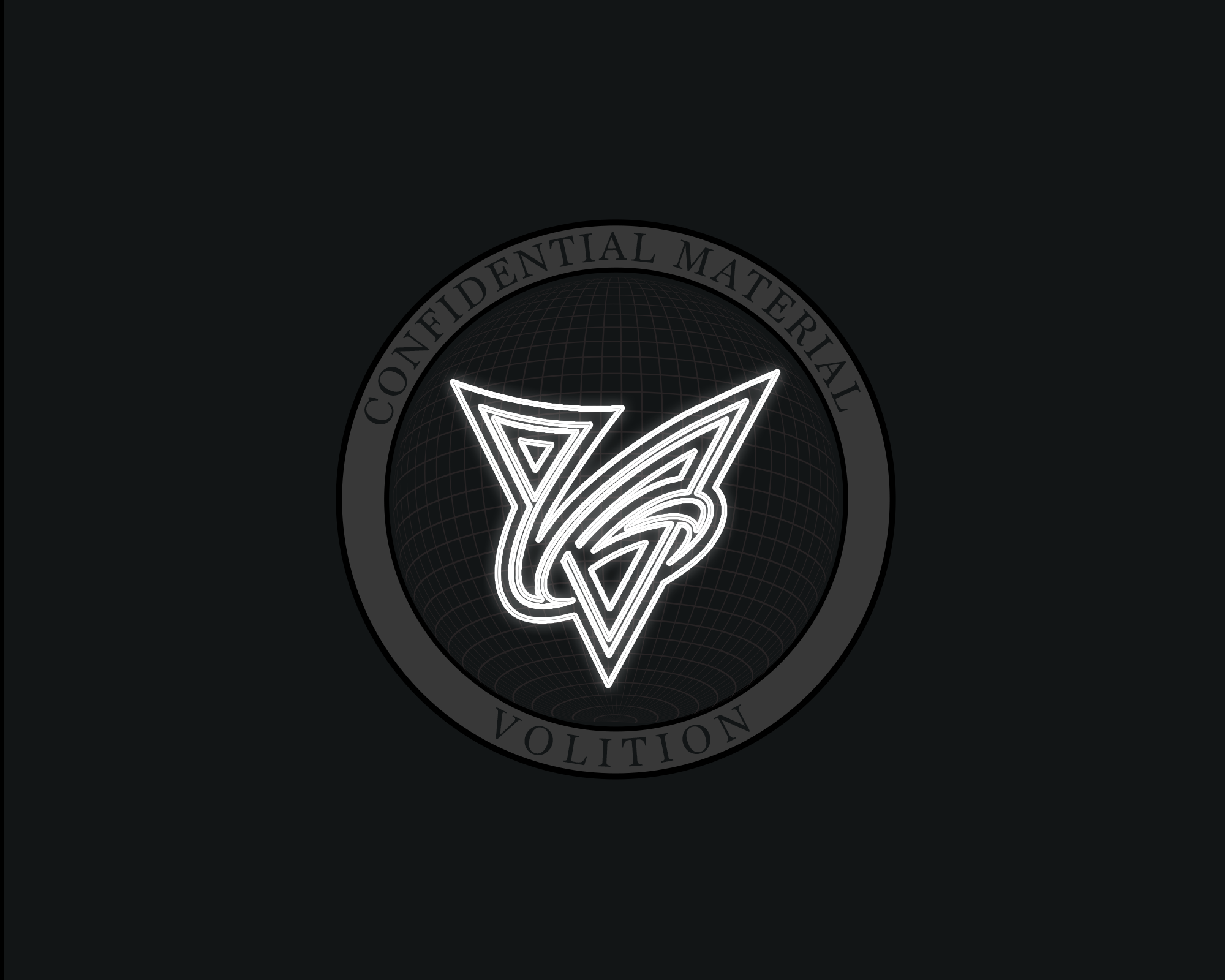